JQuery Cheatsheet
This site is a reference for JQuery
JQuery is a popular free and open-source JavaScript library used for the front-end in web applications. It is designed to simplify HTML DOM and tree traversel and manipulation, as well as event handling, CSS animation and Ajax.
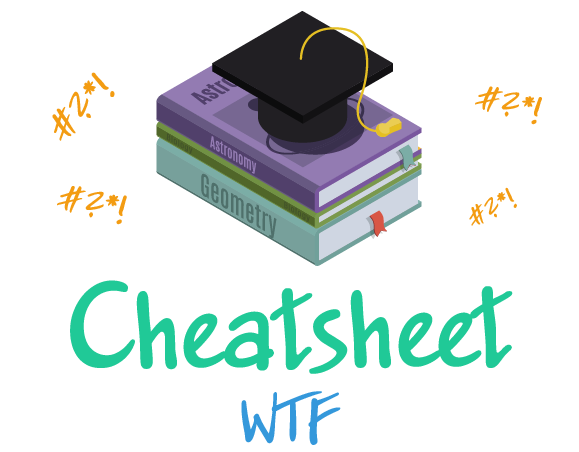
Attribute Selectors
Form Selectors
Form selectors. Used to select form elements.
Basic Selectors
Elementary JQuery selectors
Basic Filter Selectors
Child Filter Selectors
Content Filter Selectors
Hierarchy Selectors
Custom Effects
Attributes
CSS Attributes
Dimension Attributes
Offset Attributes
Data Attributes
Utilities
DOM Insertion (Around)
DOM Insertion (Inside)
DOM Insertion (Outside)
DOM Removal
Used to manipulate DOM elements from a selection of matched elements
Global Ajax Event Handlers
Ajax Helper Functions
Ajax Shorthand Methods
Filter Traversing
Used to filter traversed DOM elements that has been matched
Miscellaneous Traversing
Tree Traversal
Used to traverse the DOM tree
jQuery Core Object
DOM Element Methods
Core Internals
Deferred Object
Callbacks Object
Event Object
Mouse Events
Used to bind an event handler to mouse clicks, hovering and focusing on elements
Browser Events
Document Loading
Used to bind event handlers to be fired upon loading the document f.e.
Event Handler Attachment
Used to attach event handlers
Form Events
Used to bind event handlers to form elements
Keyboard Events
Used to bind event handlers to keyboard events such as pressing or releasing a key