Bash Cheatsheet
This site is a reference for the scripting language Bash
Bash (Bourne Again Shell) is a shell language build on-top of the orignal Bourne Shell which was distributed with V7 Unix in 1979 and became the standard for writing shell scripts. Today it is primary to most Linux distributions, MacOS and it has even recently been enabled to run on Windows through something called WSL (Windows Subsystem for Linux).
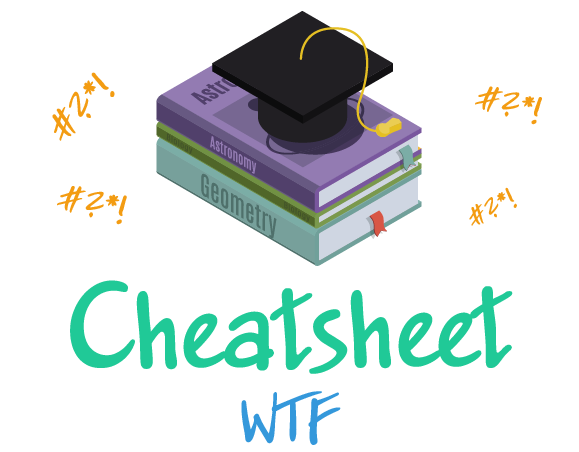
Used for testing of files files in shellscripts
Testing files in scripts is easy and straight forward. This is where shell scripting starts to show its glory! In Bash you can do file testing for permissions, size, date, filetype or existence.
Used for string comparison in Bash
This section describes how to compare strings in Bash.
Used for comparing numbers
How to compare integers or arithmetic expressions in shell scripts.
Job control allows you to selectively stop (suspend) the execution of processes and continue their execution at a later point in time.
System V Signals
UNIX System V Signals.
Useful for debugging a script. Exit takes integer args in the range 0-255.
You can use these key-combinations to send signals or control the output. Check your stty settings. Suspend and resume of output is usually disabled if you are using "modern" terminal emulations. The standard xterm supports Ctrl+S and Ctrl+Q by default.
Keyboard Shortcuts
Shortcuts to use in the CLI for swiftly editing a command. These shortcuts are provided by the GNU Readline library.
Now you may know what that arcane looking string rwxrwxrwx is when you invoke ls -l
Bash supports a surprisingly big number of string operations! Unfortunately, these tools lack a unified focus. Some are a subset of parameter substitution, and others fall under the functionality of the UNIX expr command. This results in inconsistent command syntax and overlap of functionality.
The following text shows characters that need to be quoted if you want to use their literal symbols and not their special meaning.
Command parameters, also known as arguments, are used when invoking a Bash script.
Enables use and manipulation of previous commands.
Parameter Expansions
Bash cannot recognize RegEx but understand globbing. Globbing is done to filenames by the shell while RegEx is used for searching text.
A character class is a pattern you can use instead of a regular expression. They are easier to remember and work with.
Flow control structures in Bash are straight forward, albeit Bash is unforgiving if you get the syntax wrong.
Here we have gathered a collection of all arcane syntax along with a brief description. A bunch of these symbols are repeated from earlier but many are new - this is a good starting point if you are new to the language.
Shell builins are built into Bash are often very (if not extremely) fast compared to external programs. Some of the builtins are inherited from the Bourne Shell (sh) — these inherited commands will also work in the original Bourne Shell.