Bootstrap 4 Cheatsheet
This site is a reference for Bootstrap
Bootstrap is a high-level CSS framework directed at responsive frontend development. It is amongst the most starred projects at Github with more than 140k stars.
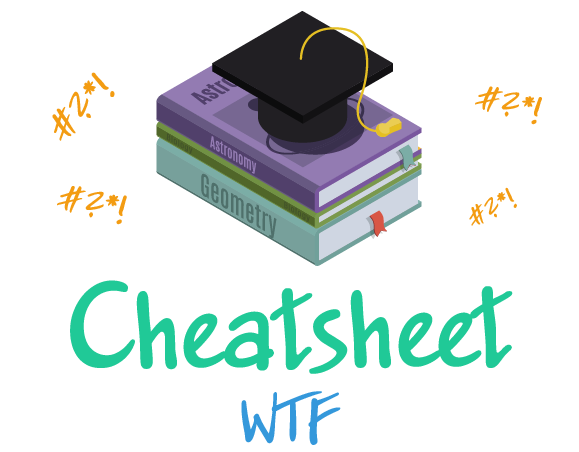
For the full experience we recommend viewing this website on a desktop or tablet.
OpSec OSINT Git Python Bash Linux CLI Package Management Regular Expressions Nano Vim HTML5 Markdown Bootstrap 4 JQuery Flask ASCII IP HTTP Port Numbers Save App
See Similar
Select a Category
View all Cheatsheets General Purpose (1) Domain Specific (1) Imperative (1) Object-Oriented (1) Web Development (3) DevOps (1) Control Versioning System (1) System Administration (2) Scripting Language (1) Compiled Language (1) Markup Language (2) Command Language (1) Framework (2) Frontend (1) Backend (6) Software Library (3) Tool (2) Standard (1) Text Editor (3) Text Processing (4)
Bootstrap 4 Categories
Use the compass to jump to a specific section of the selected cheatsheet
Notation
On The Use of Asterisks (*)
Whenever you see a class like .float-*-* the first asterisk is a replacement a class specifier (e.g. left) and the second is a breakpoint specifier (e.g. md). In action this is the same as float-<direction>-<breakpoint> e.g. float-right-lg
Copy this starter template and use it as a basis to get going instantly
<!DOCTYPE html>
<html lang="en">
<head> <!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"><!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta/css/bootstrap.min.css"> <!-- Main CSS -->
<link rel="stylesheet" href="css/main.css">
</head>
<body>
<div class="container"><h1>Hello, world!</h1>
<div class="row">
<div class="col-sm-6">Left Column</div>
<div class="col-sm-6">Right Column</div>
</div>
</div><!-- jQuery first, then Tether, then Bootstrap JS. -->
<script src="https://code.jquery.com/jquery-3.2.1.slim.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.11.0/umd/popper.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta/js/bootstrap.min.js"></script> <!-- Main JS -->
<script src="js/main.js"></script>
</body>
</html>
Use this navbar as a starting point
<!-- Button trigger modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#myModal"> Launch demo modal</button><!-- Modal -->
<div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Modal title</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body"><h2>Modal body heading</h2>
<p>Modal body text description</p>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
<button type="button" class="btn btn-primary">Save changes</button>
</div>
</div>
</div>
</div>
Use this navbar as a starting point
<form>
<div class="form-group">
<label for="exampleInputEmail1">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp" placeholder="Enter email">
<small id="emailHelp" class="form-text text-muted">We'll never share your email with anyone else.</small>
</div>
<div class="form-group"><label for="exampleInputPassword1">Password</label>
<input type="password" class="form-control" id="exampleInputPassword1" placeholder="Password">
</div>
<div class="checkbox">
<label><input type="checkbox"> Check me out </label>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
<div id="carousel-name" class="carousel slide" data-ride="carousel">
<ol class="carousel-indicators">
<li data-target="#carousel-name" data-slide-to="0" class="active"></li>
<li data-target="#carousel-name" data-slide-to="1"></li>
<li data-target="#carousel-name" data-slide-to="2"></li>
</ol>
<div class="carousel-inner" role="listbox">
<div class="carousel-item active">
<img class="d-block img-fluid" src="https://dummyimage.com/900x340/563d7c/fff&text=+" alt="First slide">
<div class="carousel-caption d-none d-md-block">
<h3>Carousel Slider Title</h3>
<p>Description text to further describe the content of the slide image</p>
<a href="" class="btn btn-primary">Call to Action</a>
</div>
</div>
<div class="carousel-item">
<img class="d-block img-fluid" src="https://dummyimage.com/900x340/563d7c/fff&text=+" alt="Third slide">
<div class="carousel-caption d-none d-md-block">
<a href="" class="btn btn-primary">Call to Action</a>
</div>
</div>
</div>
<a class="carousel-control-prev" href="#carousel-name" role="button" data-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="sr-only">Previous</span>
</a>
<a class="carousel-control-next" href="#carousel-name" role="button" data-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="sr-only">Next</span>
</a>
</div>
<div class="embed-responsive embed-responsive-16by9">
<iframe class="embed-responsive-item" src="..."></iframe>
</div>
<table class="table">
<thead class="thead-default">
<tr>
<th>#</th>
<th>table head-default</th>
</tr>
</thead>
<tbody>
<tr>
<th scope="row">1</th>
<td>Nina</td>
</tr>
</tbody>
</table>
<table class="table">
<thead class="thead-inverse">
<tr>
<th>#</th>
<th>thead-inverse</th>
</tr>
</thead>
<tbody>
<tr>
<th scope="row">1</th>
<td>Nina</td>
</tr>
</tbody>
</table>
<div class="card" style="width: 20rem;">
<img class="card-img-top w-100" src="https://dummyimage.com/600x400/563d7c/fff" alt="Card image cap">
<div class="card-body">
<h4 class="card-title">Card title</h4>
<p class="card-text">Some quick example text to build on the card title and make up the bulk of the card's content.</p>
<a href="#" class="btn btn-primary">Go somewhere</a>
</div>
</div>