Flask Cheatsheet
This site is a reference for Flask
Flask is a popular micro framework written in Python and used for building web applications. It supports extensions that can add application features as if they were implemented in Flask itself.
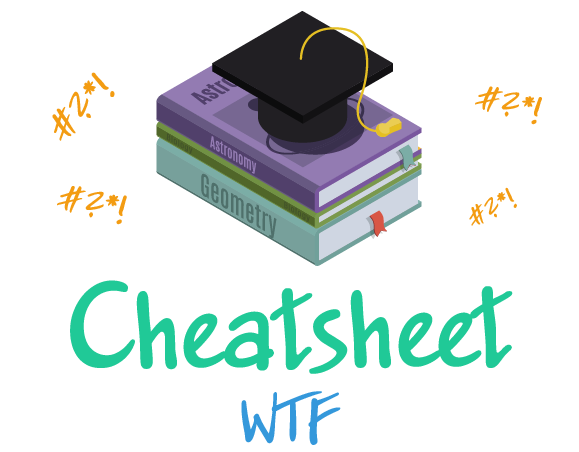
For the full experience we recommend viewing this website on a desktop or tablet.
OpSec OSINT Git Python Bash Linux CLI Package Management Regular Expressions Nano Vim HTML5 Markdown Bootstrap 4 JQuery Flask ASCII IP HTTP Port Numbers Save App
See Similar
Select a Category
View all Cheatsheets General Purpose (1) Domain Specific (1) Imperative (1) Object-Oriented (1) Web Development (3) DevOps (1) Control Versioning System (1) System Administration (2) Scripting Language (1) Compiled Language (1) Markup Language (2) Command Language (1) Framework (2) Frontend (1) Backend (6) Software Library (3) Tool (2) Standard (1) Text Editor (3) Text Processing (4)
Flask Categories
Use the compass to jump to a specific section of the selected cheatsheet
This example shows you how the minimal code required to setup a functional Flask app
from flask import Flask
app = Flask(__name__)
# Make a route accessible, i.e. www.example.com/hello-world/
@app.route('/hello-world/')
def hello():
# Method which is executed when user accesses www.example.com/hello-world/
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
@app.route('/<string:name>/<int:age>')
def hello(name, age):
return 'Hello ' + name + ', ' + 'you are ' + age + ' years old!'
# Other route converters
# string -> The default. Accepts any text without a slash.
# float -> A floating point integer, i.e. 4.32137
# int -> An integer, i.e. 7
# path -> Like a string but also accepts slash.
# uuid -> Accepts UUIDs (universally unique identifiers); think hexcode on steroids.
@app.route('/test') # Accepts 'GET' by default and no other.
@app.route('/test', methods=['POST']) # Allows only 'POST'.
@app.route('/test', methods=['POST','PUT']) # Allows 'PUT' and 'POST' and no other.
app.config['CONFIG_NAME'] = 'config value' # E.g. app.config['SECRET_KEY'] = '832dcb4c00910ad5de1db300488e26d9'
app.config.from_envvar('ENV_VAR_NAME') # Gets the value from an environment value. Useful for API keys and similar information.
from flask import render_template
@app.route('/')def index():
return render_template('template_file.html', var1=value1, ...) # Specify as many vars as you want to, to pass down to the template.
import jsonify
@app.route('/returnstuff')
def returnstuff():
num_list = [1,2,3,4,5]
num_dict = {'numbers' : num_list, 'name' : 'Numbers'}
return jsonify({'output' : num_dict})
# Usefull if you f.e. want to create a REST API for connecting the backend to the frontend.
request.args['name'] # Query string arguments
request.form['name'] # Form data
request.method # Used to determine the request type. Can be used to check if user is POST'ing, PUT'ting, GET'ting etc.
request.cookies.get('cookie_name') # Getting a cookie, by name 'cookie_name'
request.files['name'] # Retrieving a file
from flask import url_for, redirect
@app.route('/home')
def home():
return render_template('home.html')
@app.route('/redirect')
def redirect_example():
return redirect(url_for('home')) # Redirects the user to /home
# url_for('') retrieves route and method for whatever method you give it.
from flask import abort()
@app.route('/')
def index():
abort(404) # Returns 404 error. Use the errorhandler below to catch http codes, i.e. redirecting to your own 404 page.
render_template('index.html') # This never gets executed
@app.errorhandler(404)
def page_not_found(error):
return render_template('404.html', title='404: Missing Page'), 404
import session
app.config['SECRET_KEY'] = 'any random string' # Secret key must always be set to use sessions
# Set a session
@app.route('/login_success')
def login_success():
session['key_name'] = 'key_value' #stores a secure cookie in browser
return redirect(url_for('index'))
# Read a session
@app.route('/')
def index():
if 'key_name' in session: # Session exists and has key named 'key_name'
session_var = session['key_value']
Heavily inspired by Pretty Printed Flask Cheatsheet